# 一、Redux 认知
# 1、纯函数
简单的说就是,有确定的输入/输出、函数执行过程中不能产生副作用
在 react 官方文档的 Props 的只读性 (opens new window) 中也有“纯函数”的相关描述:
所有 React 组件都必须像纯函数一样保护它们的 props 不被更改。
提示
在 redux 中,reducer 同样别要求必须是一个纯函数。
# 2、Redux 产生
在 React 中,react 主要负责帮我们管理视图,state 最终是由开发者自己维护的
UI = render(state)
Redux 就是一个帮助我们管理 State 的容器
2015 年,Redux 出现,将 Flux 与函数式编程结合一起,很短时间内就成为了最热门的前端架构。
# 3、三大原则
- 单一数据源
其实,Redux 中并没有强制让我们不能创建多个 Store
- State 是只读的
触发 action 是修改 State 的唯一方法
- 纯函数执行修改
reducer 可以将旧 state 和 action 建立联系,并返回一个新的 State(没有修改原 State,而是返回一个新的 State)
# 二、Redux 使用
-Redux (opens new window) 是 JavaScript 应用程序的可预测状态容器。
安装:
npm install redux
# 1、简单示例
- 构建 store:
// store / index.js
import { createStore } from 'redux'
// state初始化值
const initialState = {
//
count: 6,
name: 'ren'
}
// reducer函数(纯函数)
function reducer(state = initialState, action) {
// console.log(state, action)
// actions处理
switch (action.type) {
case 'count_increment':
return { ...state, count: state.count + 1 }
case 'name_change':
return { ...state, name: action.name } // 在没有修改原state的前提下,返回了新的state
default:
return state
}
//
// return state
}
// 大佬store产生了
export default createStore(reducer)
- 使用 Redux:
import store from '@/store/index.js'
// 1、监听state(初始化)值
// 手动
const state = store.getState()
console.log(state)
// 自动
const unsubscribe = store.subscribe(() => console.log(store.getState())) // 启用
unsubscribe() // 取消
// 2、修改state(初始化)值
store.dispatch({ type: 'count_increment' })
store.dispatch({ type: 'name_change', name: 'lencamo' }) // 传值
# 2、store 拆分 ✨
目录结构:
这里并不是模块化,而仅仅是对某个 store 进行了拆分
├── store
├── index.js # store
├── constants.js # 保证action命名一致
├── reducer.js # reducer逻辑抽离
└── actionCreators.js # action使用封装
文件内容:
- index.js
import { createStore } from 'redux'
import reducer = require("./reducer.js")
export default createStore(reducer)
- constants.js
export const COUNT_INCREMENT = 'count_increment'
export const NAME_CHANGE = 'name_change'
- reducer.js
import { COUNT_INCREMENT, NAME_CHANGE } from './constants.js'
const initialState = {
count: 6,
name: 'ren'
}
function reducer(state = initialState, action) {
switch (action.type) {
case 'COUNT_INCREMENT':
return { ...state, count: state.count + 1 }
case 'NAME_CHANGE':
return { ...state, name: action.name }
default:
return state
}
}
export default reducer
- actionCreators.js
import { COUNT_INCREMENT, NAME_CHANGE } from './constants.js'
export const countIncrementAction = () => ({
type: COUNT_INCREMENT
})
export const nameChangeAction = (name) => ({
type: NAME_CHANGE,
name
})
- 使用 Redux
import store from '@/store/index.js'
import { countIncrementAction, nameChangeAction } from './actionCreators.js'
store.dispatch(countIncrementAction())
store.dispatch(nameChangeAction('lencamo'))
# 3、工作流程
官方:
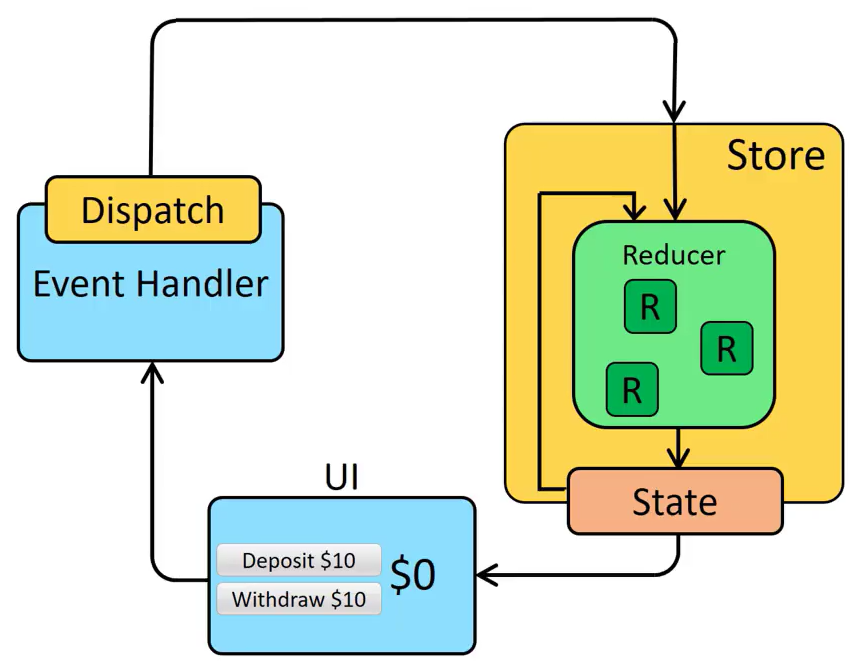
其他流程图
- 阮一峰
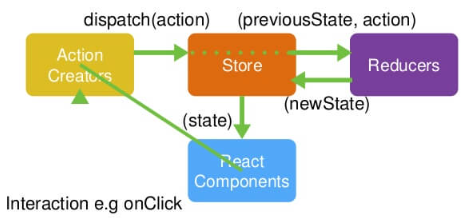
- codewhy
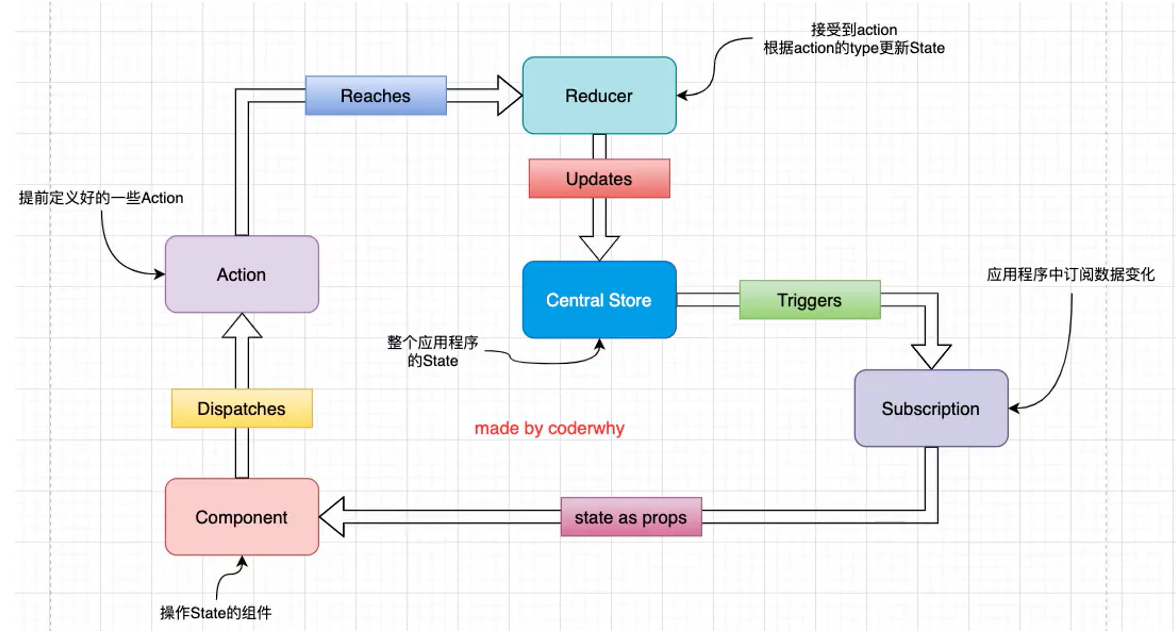
← 自定义Hooks React-Redux →