# 一、package.json
下载任意一个 npm 包后,项目文件会自动生成如下的文件
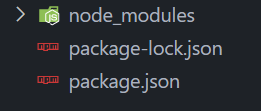
由于项目的包体积较大,不利于项目源码共享,所以要将node_modules
文件夹添加到 Git 的.gitignore
忽略文件中
关于忽略文件,详细内容可以参考git 文档 (opens new window)
# 1、版本号 ✨
关于 package.json 中包的版本号前缀标识符 version:
提示
npm 包的版本通常是需要遵从 semver 版本规范 (opens new window)的。
包的版本号是以“点分十进制”形式进行定义的
# X:重大版本更新(可以参考vue2 --> vue3)
# Y:新功能、向下兼容
# Z:修复版本的bug
X.Y.Z
① 范围:
- 任意版本
*
:对版本没有限制(一般不用) - 对比符号类的:>、>=、<、<=
- 或符号
||
:可以用来设置多个版本号限制规则,例如 >= 3.0.0 || <= 1.0.0
② 固定:
- 插入符号
^
:固定主版本号(默认方式) - 波浪符号
~
:固定主版本号和次版本号 - 没有任何符号:必须使用当前版本号(=)
# 2、脚本
{
"scripts": {
// 可以直接使用 npm run start
"start": "node ./src/main.js"
}
}
# 3、证书
licence 略
# 二、npm 包
# 1、基本要求
- 单独 存在于一个文件夹中
- 顶级目录下必须包含 package.json
- package.json 中必须包含 name、version、main 三个属性
当然 package.json 通常使用 npm init -y 自动生成
# 2、目录结构
使用 require 调用包时,会首先找到包文件夹中的 package.json 中的 main 属性,然后转到相应的入口文件中
renutil
├─ src (核心功能)
| ├─ dateFormat.js
| └─ htmlEscape.js
├─ index.js (入口文件)
├─ package.json
└─ README.md
# 三、开发 npm 包
# 1、包开发
开发个人包示例
- index.js
// index.js文件为入口文件
// 导入模块化拆分的js文件
const date = require('./src/dateFormat')
const escape = require('./src/htmlEscape')
// 共享模块作用域
// module.exports = {
// dateFormat,
// htmlEscape,
// htmlUnEscape,
// };
// 使用es6语法:—— 展开🚩运算符
module.exports = {
...date,
...escape
}
- package.json
{
"name": "renutil",
"version": "1.0.0",
"main": "index.js",
"description": "格式化时间,HTML Escape功能",
"keywords": ["lencamo", "dateFormat", "escape"],
"license": "ISC",
"homepage": "官网地址",
"repository": {
"type": "git",
"url": "包github地址"
}
}
- src/dateFormat.js
// 一、格式化时间
function dateFormat(dtStr) {
// 这个注意区别new Date()
const dt = new Date(dtStr)
const y = dt.getFullYear()
const m = fixZero(dt.getMonth() + 1)
const d = fixZero(dt.getDate())
const hh = fixZero(dt.getHours())
const mm = fixZero(dt.getMinutes())
const ss = fixZero(dt.getSeconds())
// 使用【模块🚩字符串】 来拼接
// return `YYYY-MM-DD hh:mm:ss`;
return `${y}-${m}-${d} ${hh}:${mm}:${ss}`
}
// 自定义补零函数
function fixZero(n) {
return n > 9 ? n : '0' + n
}
// 共享模块作用域
module.exports = {
dateFormat
}
- src/htmlEscape.js
// 二、HTML Escape功能
function htmlEscape(htmlstr) {
return htmlstr.replace(/<|>|"|&/g, (match) => {
switch (match) {
case '<':
return '<'
case '>':
return '>'
case '"':
return '"'
case '&':
return '&'
}
})
}
function htmlUnEscape(str) {
return str.replace(/<|>|"|&/g, (match) => {
switch (match) {
case '<':
return '<'
case '>':
return '>'
case '"':
return '"'
case '&':
return '&'
}
})
}
// 共享模块作用域
module.exports = {
htmlEscape,
htmlUnEscape
}
# 2、包发布
发布包之前要先注册 npm 账号 (opens new window)。发布包时,要在包的根目录下进行。
cd [包路径]
# nrm ls
# nrm use npm
npm login
# 包发布
npm publish
# 3、包删除
Refusing to delete the last version of the package. It will block from republishing a new version for 24 hours
# 包删除
npm unpublish [email protected] --force # --force强制删除
# 【默认情况下同一个包,其最后一个版本不可以直接删除,并且 24小时内不可以发布下一个版本】